Running Source Code with the Code Editor
Learn how to run source code snippets on Signaloid's uncertainty-tracking processors.
The Signaloid Cloud Developer Platform offers you a way to directly run source code on Signaloid uncertainty-tracking C0 processors using the Code Editor (Figure 1).
The Code Editor page contains the Editor, the Result Panel, and Variable Viewer:
- Editor—allows you to directly write code and run it on the Signaloid Cloud Compute Engine. The Editor contains controls to specify the Core to run on and the command line arguments for the program, to configure Data Sources, and theme () and layout controls ( or ).
- Result Panel—has tabs that show the program outputs and other runtime information after executing the program.
- Variable Viewer—allows to discover and select variables to trace during execution of the program on a Core with the Reference microarchitecture. The Variable Viewer is hidden by default: toggle it with the button.
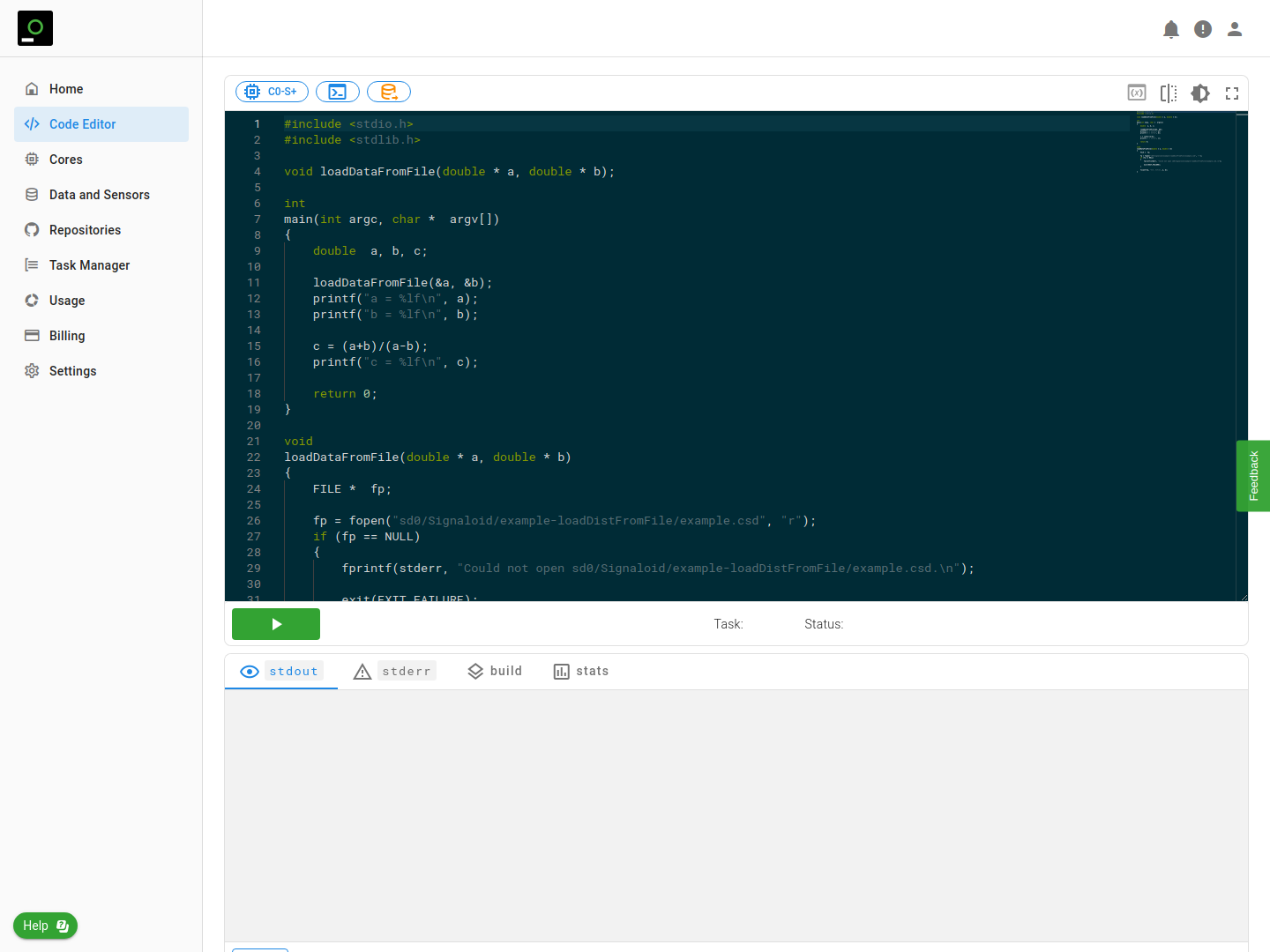
Running a program
When you first sign in, the Editor contains a simple program that reads data from a file into two variables (a
and
b
), performs a calculation, and prints the result (c
).
The default program assumes the active mount configuration is the platform-default mount configuration for Data
Sources. You can change or reset the active mount configuration with the button. The platform-default mount configuration for Data Sources mounts your Signaloid Cloud Storage home directory to sd0/
.
The default program:
#include <stdio.h>
#include <stdlib.h>
void loadDataFromFile(double * a, double * b);
int
main(int argc, char * argv[])
{
double a, b, c;
loadDataFromFile(&a, &b);
printf("a = %lf\n", a);
printf("b = %lf\n", b);
c = (a+b)/(a-b);
printf("c = %lf\n", c);
return 0;
}
void
loadDataFromFile(double * a, double * b)
{
FILE * fp;
fp = fopen("sd0/Signaloid/example-loadDistFromFile/example.csd", "r");
if (fp == NULL)
{
fprintf(stderr, "Could not open sd0/Signaloid/example-loadDistFromFile/example.csd.\n");
exit(EXIT_FAILURE);
}
fscanf(fp, "%lf, %lf\n", a, b);
}
Starting a Task
A Task is a single execution of an application on the Signaloid Cloud Developer Platform or the Signaloid Cloud Compute Engine.
Every program execution on the Signaloid Cloud Developer Platform is called a Task and the Compute Engine assigns it a Task ID: a unique string that globally identifies the Task.
Click the button to execute the program. The
Editor displays the Task ID and the Task status next to the icon. When the Task status becomes Completed
it means the
program finished execution successfully. The Result Panel will
contain the program outputs. Figure 2 shows the state of the page after the Task has completed execution.
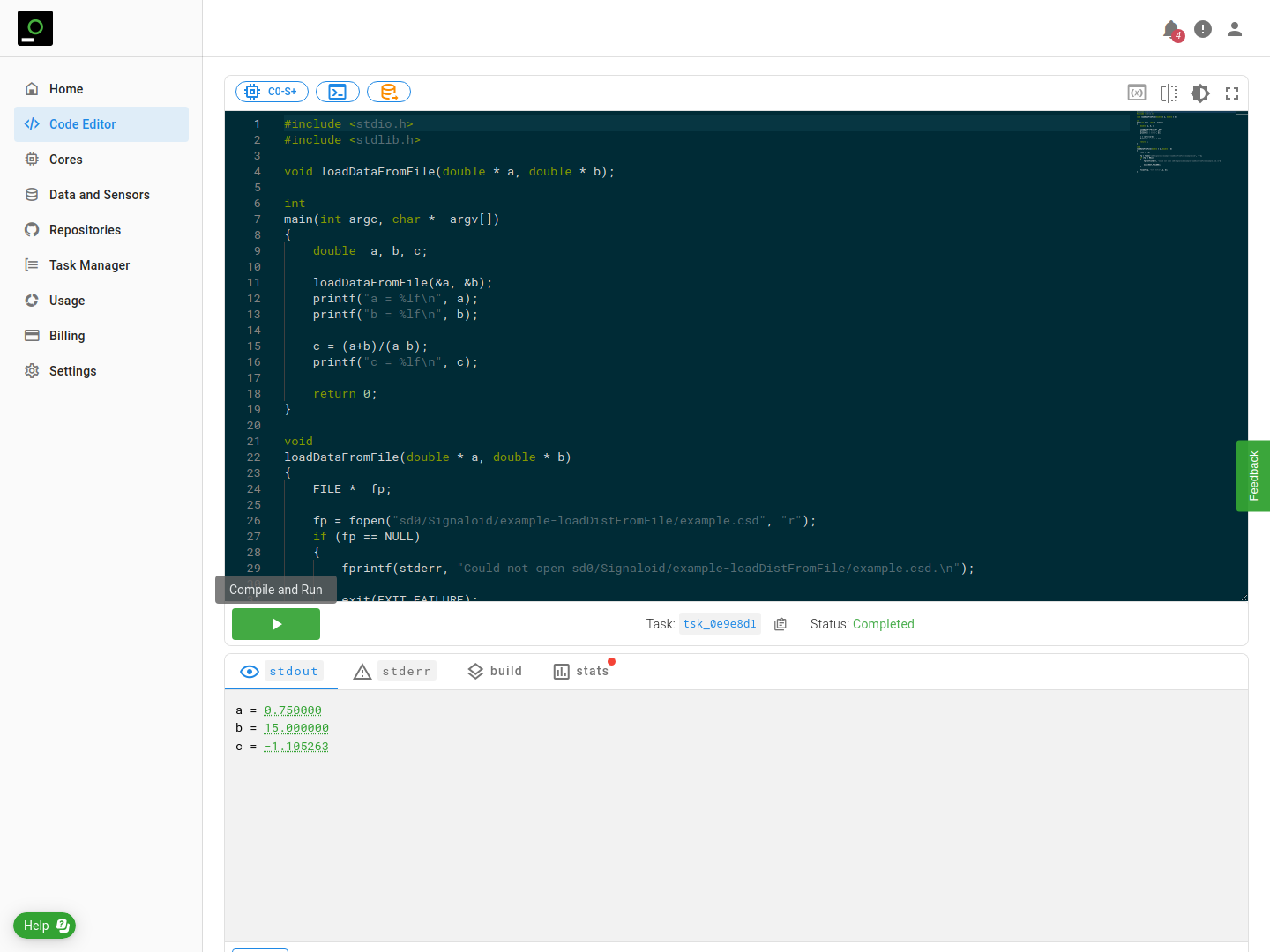
Program output and the Result Panel
The program prints the two inputs a
and b
, and the output c
. By default, the Result
Panel shows the stdout
, the stderr
, the build, and the runtime
information tabs:
- The
stdout
tab is where prints to the program's standard output went. The Signaloid C0 uncertainty-tracking processors track variable value uncertainty. Printed variables that have associated uncertainty are in underlined and in green typeface. Hovering over these values or clicking them shows a plot of the uncertainty associated with the value in the form of a histogram. - The
stderr
tab shows the program output written to the standard error file descriptor. - The build tab shows errors and warnings from the build process when relevant.
- The runtime information tab shows system information about the executed Task, such as the actual amount of execution time on the C0 processor and the total dynamic instruction credits the Task consumed.
Changing the layout
The Code Editor has two settings that affect the layout switches: the Toggle Layout button and the Toggle Variable Viewer button (learn more about the Variable Viewer). The Toggle Layout button icon changes between and depending on the current layout.
Setting command-line arguments
The Signaloid Cloud Development Platform enables you to set command-line arguments for your program via a dialog that opens by clicking the Command Line Arguments button. The platform will pass the command-line arguments specified in the text area to your application when you run it.
The platform stores these command-line arguments and uses them in all follow-up Task executions from the Code Editor page. To reset the command-line arguments, open the dialog again, remove your entries from the text area, and press the Set Arguments button.
The arguments are available to your application via the common arguments interface for your programming language. For
example, for Tasks written in C, the arguments are accessible in the argv
array (of length argc
).
The Signaloid Cloud Compute Engine does not support quoting arguments that contain spaces to create a single argument.
In other words, if you set the command-line argument text area to arg1 "arg2p1 arg2p2"
, the compute engine will treat
"arg2p1
and arg2p2"
as two separate arguments.